Creating an IoT battery monitor using the ESP8266 is a popular and practical project. In this project, you can monitor the battery voltage of a device remotely using Wi-Fi and send the data to Arduino in the cloud. Here’s a simplified outline of how to create an IoT battery monitor using an ESP8266
COMPONENTS NEEDED
- ESP8266 (e.g., NodeMCU or Wemos D1 Mini)
- 2*100k Resistors (Voltage Divider Circuit to measure the battery voltage)
- tp4056 charging module
- Battery to be monitored
- Breadboard and Jumper Wires
- Access to an IoT Platform or Web Server
CIRCUIT DIAGRAM
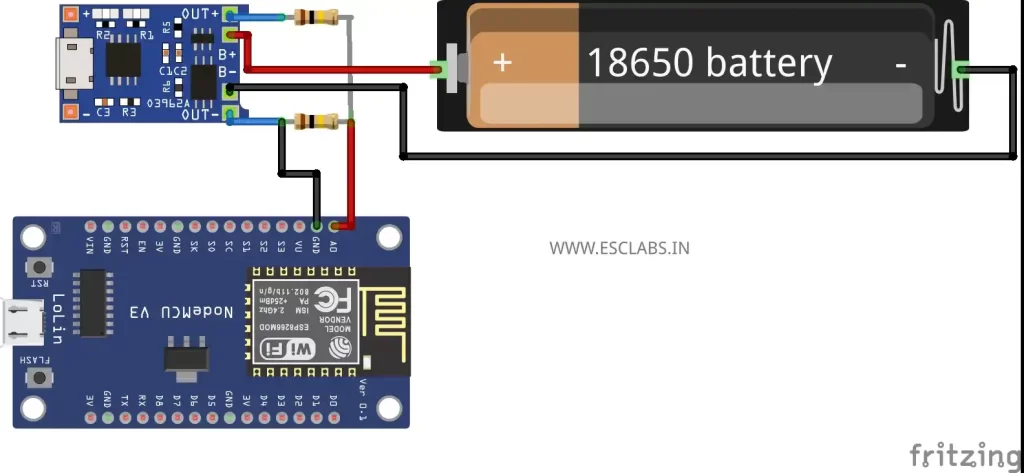
VOLTAGE DIVIDER NETWORK
A voltage divider is a simple circuit used to scale down a higher voltage to a lower voltage level, making it suitable for measurement by a microcontroller’s analog input. In the context of measuring battery voltage with an ESP8266 or similar microcontroller, you can use a voltage divider to bring the battery voltage within the safe operating range of the microcontroller’s ADC (analog-to-digital converter), typically 0-3.3V for the ESP8266.
Select the Resistance Values: Determine the voltage range you want to measure and select suitable resistor values for R1 and R2. The formula for the voltage division is:
Vout = Vin * (R2 / (R1 + R2))
Where:
Vin
is the voltage you want to monitor.Vout
is the voltage you want to read with the NodeMCU.R1
is the resistance of the first resistor.R2
is the resistance of the second resistor.
Ensure that R2 is smaller than or equal to R1 so that the output voltage doesn’t exceed the NodeMCU’s maximum analog input voltage (usually 3.3V for NodeMCU).
ARDUINO CODE
int analogInPin = A0;
int sensorValue;
float voltage;
float bat_percentage;
void setup() {
Serial.begin(9600);
delay(1500);
}
void loop() {
sensorValue = analogRead(analogInPin);
voltage = (((sensorValue * 3.3) / 1024) * 2 );
bat_percentage = mapfloat(voltage, 2.8, 4.2, 0, 100);
if (bat_percentage >= 100)
{
bat_percentage = 100;
}
if (bat_percentage <= 0)
{
bat_percentage = 1;
}
Serial.print("Analog Value = ");
Serial.print(sensorValue);
Serial.print("\t Output Voltage = ");
Serial.print(voltage);
Serial.print("\t Battery Percentage = ");
Serial.println(bat_percentage);
delay(1000);
}
float mapfloat(float x, float in_min, float in_max, float out_min, float out_max)
{
return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min;
}
Leave a comment